MongoDB – Java Spring: Part 1 ( Setup, Compass, Basic Queries)
MongoDB is one of the leading and widely used distributed No-SQL document storage engine. This is evident from the number of organizations using the service and the support available online. MongoDB stores the data in the form of documents and can be viewed in JSON format. It has also got a query language of its own and has indexing and most importantly strong aggregation support.
In this post, we will be checking out the MongoDB functionalities, document structure, Compass ( the UI tool for exploring and managing the components ) and the aggregations available.
Please note the following:
- MongoDB setup is not covered in the post
- Test data is assumed to be loaded from the Java spring application ( https://github.com/microideation/mongodb-demo ).
You can also watch the video version of this tutorial on YouTube
MongoDB
MongoDB is used by many applications to store related information as a single document. Traditional relational data-stores are mostly used to store the data in a normalized manner and need to use joins while fetching the information. MongoDB model will allow users to store the associated information a single document ( and still supports joins if required)
Setup
You can install MongoDB by going to the download page. Follow the instructions for installation based on your version and platform.
https://www.mongodb.com/download-center/community
Creating an admin user
By default, MongoDB starts without authentication and can only be connected from the localhost. To add an admin user, we need to open the mongo shell. Put the following in command line.
mongo
Put the following in the prompt to create a user with name “madmin” and password as “madmin”
db.createUser({user:"madmin",pwd:"madmin",roles:[{role:"userAdminAnyDatabase",db:"admin"}]})
Edit the /etc/mongod.conf file and change the value of security.authentication to “enabled”
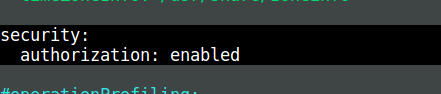
Restart MongoDB service and access the mongo shell. We can now put the following commands and authenticate using the new admin user.
use admin db.auth("madmin","madmin")
Create a user for a specific database
We can create a user specifically for a database. For eg: If we need to create a user for “in-profile-test” DB, then we do the following:
use admin db.auth("madmin","madmin") use in-profile-test db.createUser({user:"profileuser",pwd:"profileuserpwd",roles:[{role:"readWrite",db:"in-profile-test"}]})
This will create a user “profileuser” with password “profileuserpwd” having read and write permissions to “in-profile-test” DB. Note that you don’t need to create the DB beforehand.
Configuring MongoDB to be connected from other IPs
By default, MongoDB is only allowed to connect from the localhost. We can change this behavior by editing the configuration.
Edit the /etc/mongod.conf file
Under net.bindIp, set the IP addresses to which it needs to be bound with comma. Better to provide localhost also as by default the mongo will try to connect to local MongoDB.
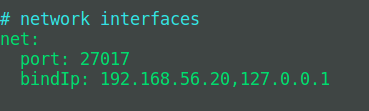
MongoDB Compass
MongoDB Compass is a GUI provided by MongoDB for exploring and managing the MongoDB documents and databases. The community edition is free to use and has most of the functionalities required for everyday use. This is a must-have tool if you are working with the MongoDB databases on a day to day basis.
Installation
You can download and install the Compass from the download center from the below link.
https://www.mongodb.com/download-center/compass
Make sure to choose the right platform applicable for your system and also choose the version as “Community Edition”. The rest of the installation process will be familiar to your respective operating system.
Connecting to DB
Compass allows connection to a self-hosted MongoDB instance or to a MongoDB Atlas URL. You can open the Compass and click on “New Connection” to setup a new connection. For a local instance, you can just provide the hostname ( or IP ) , username, password. Make sure to choose the Authentication as “SCRAM-SHA-256”
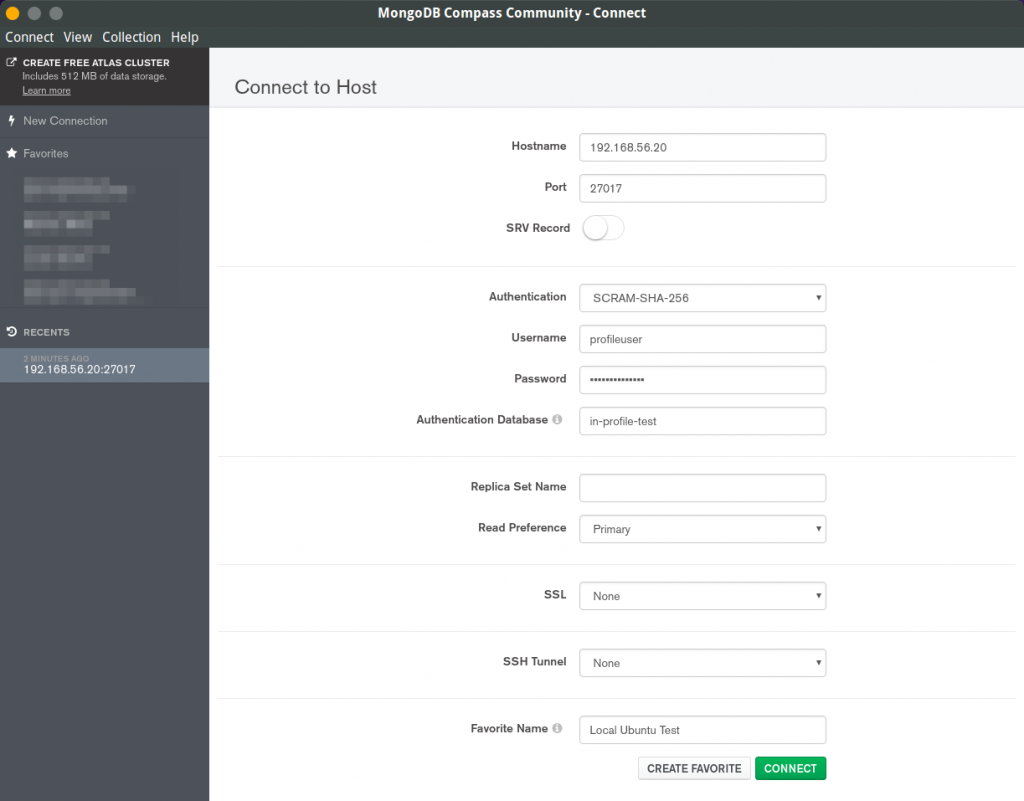
Compass Components
Compass has the following sections
- DB & Collections ( left side )
- The main section listing the collections and query results.
We can click on a collection name and it will be expanded to the sample documents in the collection. You will be able to see the query field, the result field, etc. You can also edit a particular entry field or the embedded objects of a document from within the compass.
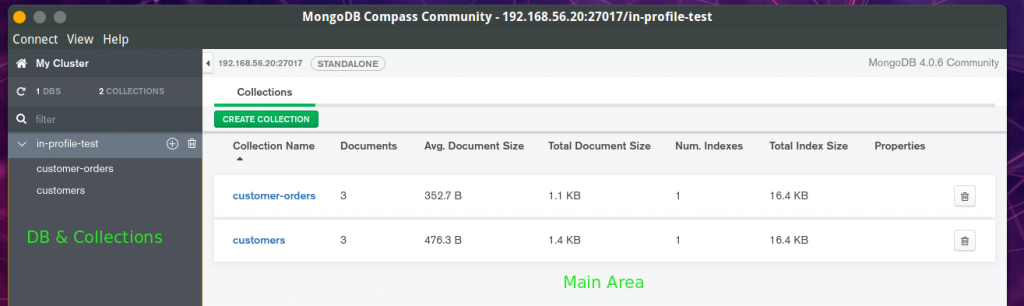
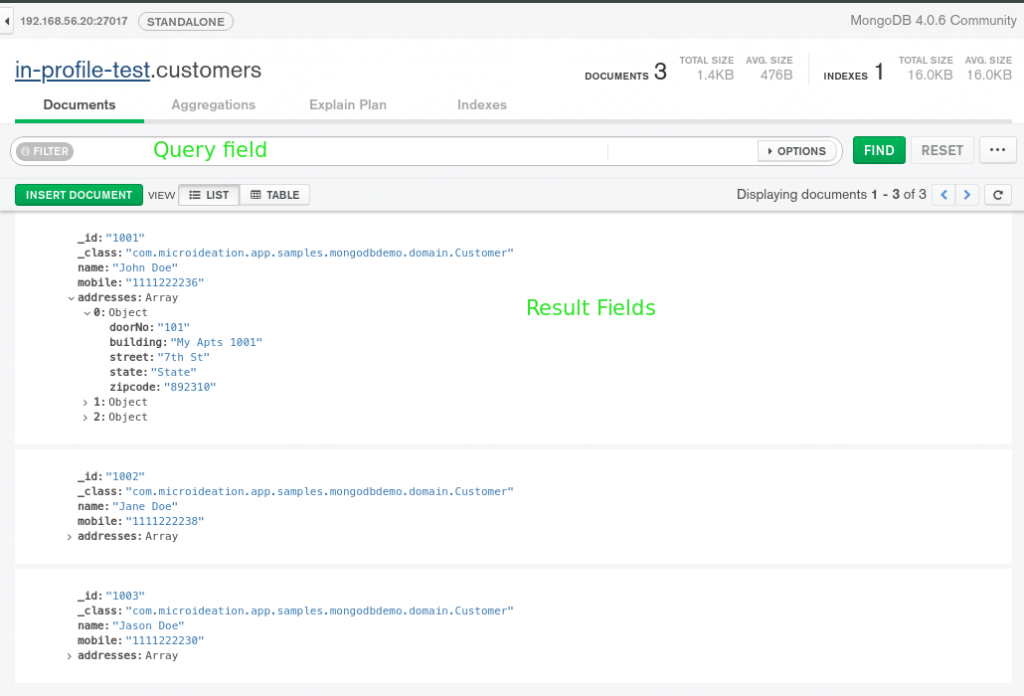
Aggregations, Indexes & More
Apart from the query and results, one major area is Aggregations in the Compass. There are powerful tools to generate complex aggregates based on the documents and embedded objects. Compass has first-class support for the aggregations and allows building a pipeline with code completion and assistance.
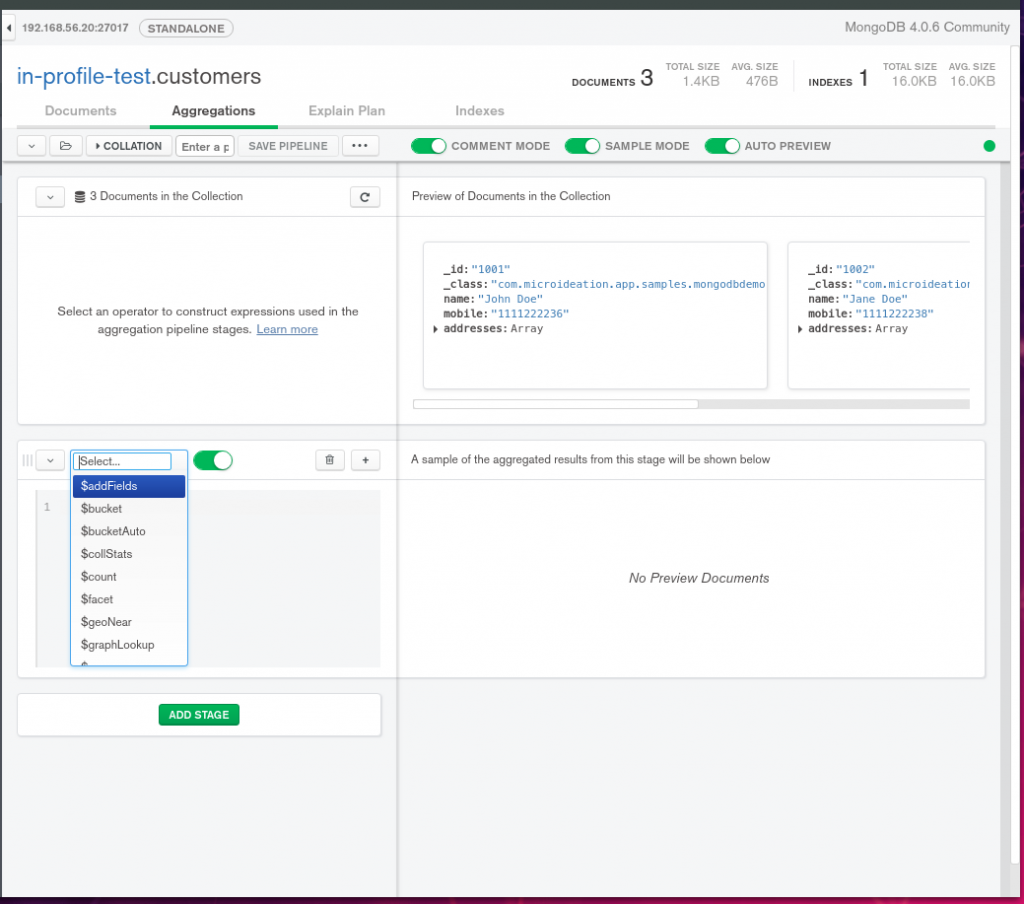
We will see aggregations and pipelines in detail on Part 2 of this tutorial.
Also, there are options to create indexes on the document fields and also to see the execution plan for a particular query based on which we can create indexes to optimize the execution time.
Below, we can see that the query on the name is not having an index and is going through all the documents.
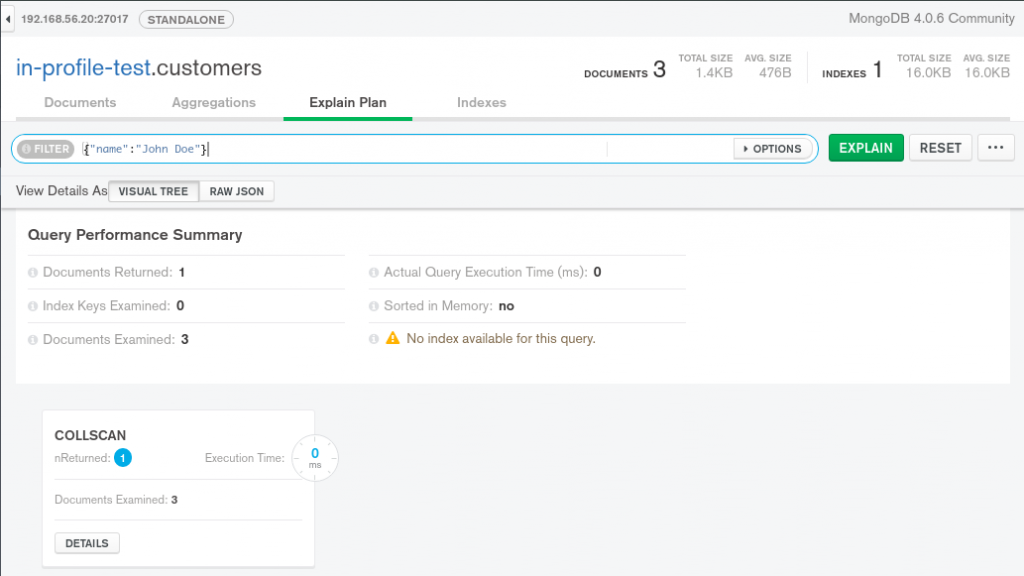
We can create an index on the name column by going to the “Indexes” section of the compass. Click on “Create Index” and fill in the details as in the screenshot below:
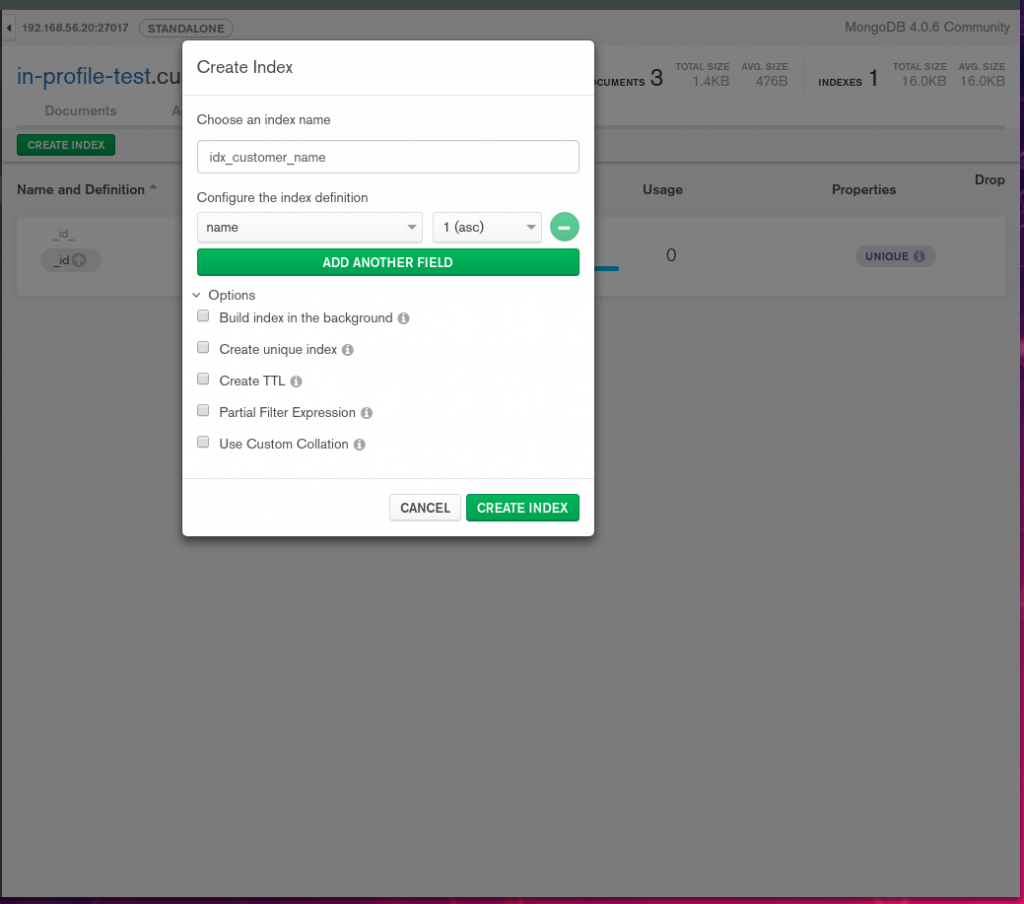
Now, let’s check the execution plan for the same query again.
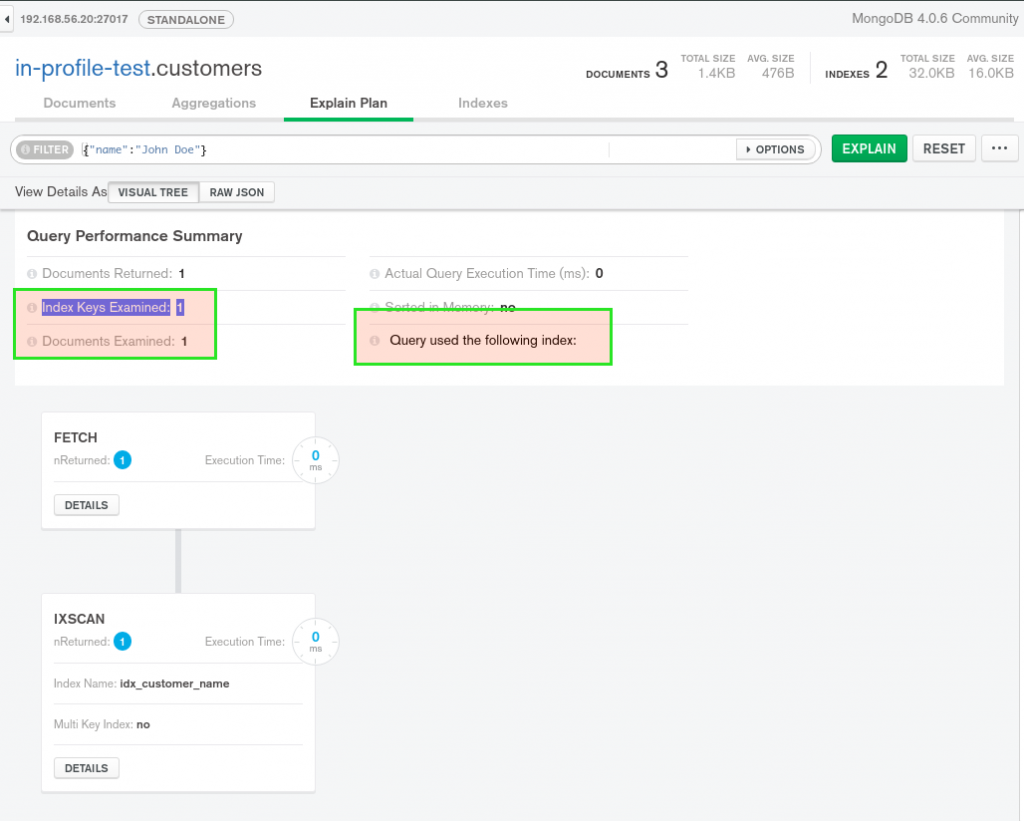
We can also create a multi-key index or complex indexes by combining different fields on the document. You can also create an index for a field in the embedded document ( For eg: In our customer example, we can create an index on the addresses. zipcode field if we are searching there frequently ).
Documents
Documents refer to the specific structure of the information we are storing. A set of documents is called a collection and is analogous to the tables in the relational DB. In this way, we can think of a document as a row of data. But here the document can have structural differences. Like in the below case, the customer is a collection and each document can have customer info and associated addresses. But we can skip any fields or add new fields to individual document unlike relational DB where the columns added are replicated across all rows.
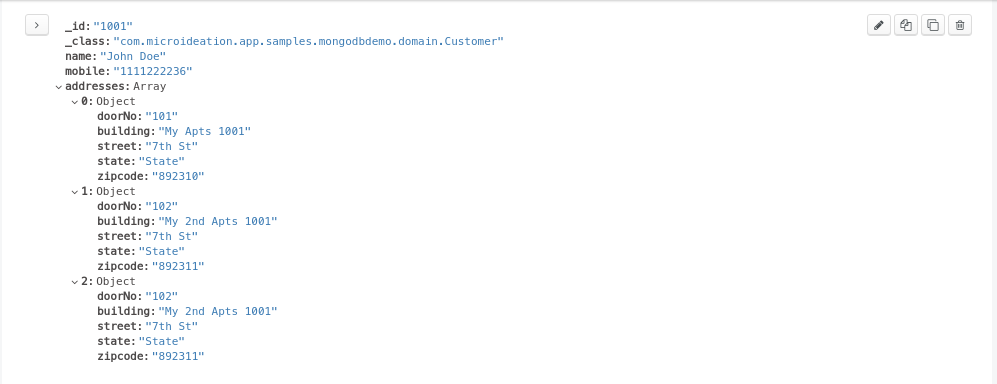
Query language & Basic queries
MongoDB supports the querying of information using json like query language. There are several functions that can be used in the language for checking equality, comparison, relational operations etc.
Basic query
For eg: To find the customers with the id “1002” in the above example, we will be writing below query
{"_id":"1002"}
For MongoDB, the id field marked will be referenced by _id . In the above, query is a simple json requesting to match the data.
Queries can be executed from the mongo command line on the database or my preferred method of using the MongoDB Compass. All the subsequent examples will be based on the MongoDB compass tool. You can download and setup from here. Make sure to choose the “Community edition”
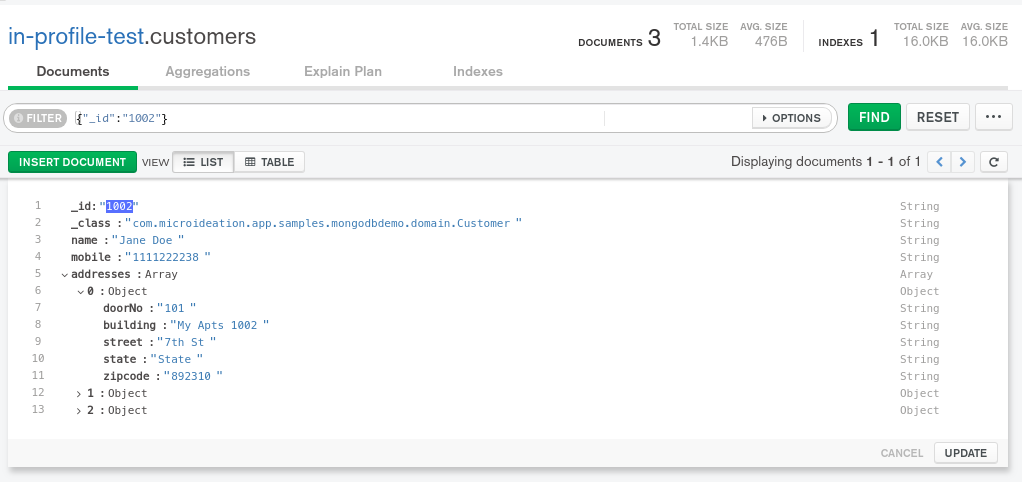
AND conditions
To query with the “AND” condition, we use the comma-separated fields in JSON. For eg: To find the customer with id “XXXX” and name “XXX”, we will use the below query
{"_id":"1002","name":"Jane Doe"}
OR conditions
To query with the “OR” condition, we need to use the MongoDB function “OR”. The MongoDB functions can be referenced in a query using the “$” symbol. The or has format as below
$or:[{expr1},{expr2}
So to find the customers whose name are either “John Doe” or “Jane Doe”, we will use the below query
{$or:[{"name":"John Doe"},{"name":"Jane Doe"}]}
Relational Operators ( lt, gt , lte, gte )
MongoDB supports relational operations using the functions lt ( less than ), gt ( greater than), lte ( less than or equal to), gte ( greater than or equal to). We can try these methods in the “customer-orders” to find the orders with a specific value
Find all orders with price LESS THAN OR EQUAL 300 - > {"price":{$lte:300}} Find all orders with price LESS THAN 300 - > {"price":{$lt:300}} Find all orders with price GREATER THAN 300 - > {"price":{$gt:300}} Find all orders with price GREATER THAN OR EQUAL 300 - > {"price":{$gte:300}}
Query on embedded objects
We can also write query on the fields of the embedded objects of a document. For eg:, if we can want to find all the customers with zipcode as a particular value, we can execute the below query
{"addresses.zipcode":"892314"}
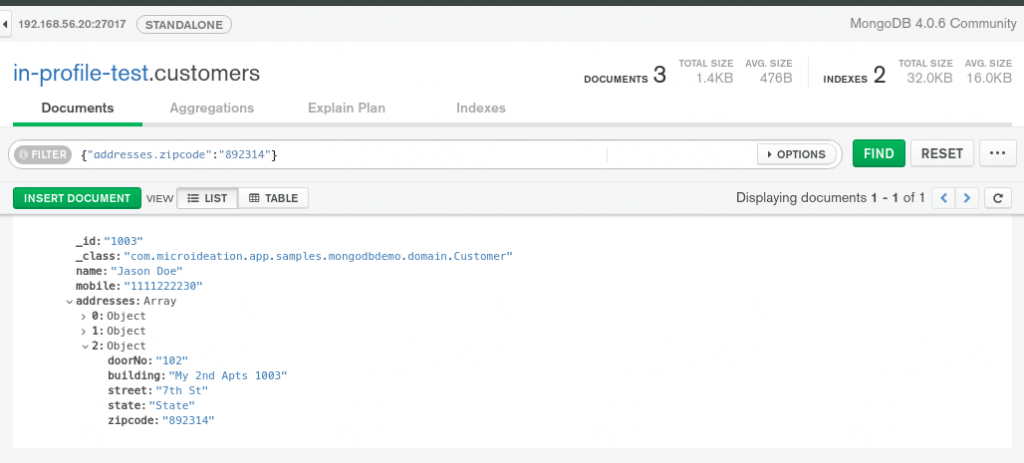
If there are muliple documents with same value for the embedded address, then all the documents matching will be returned.
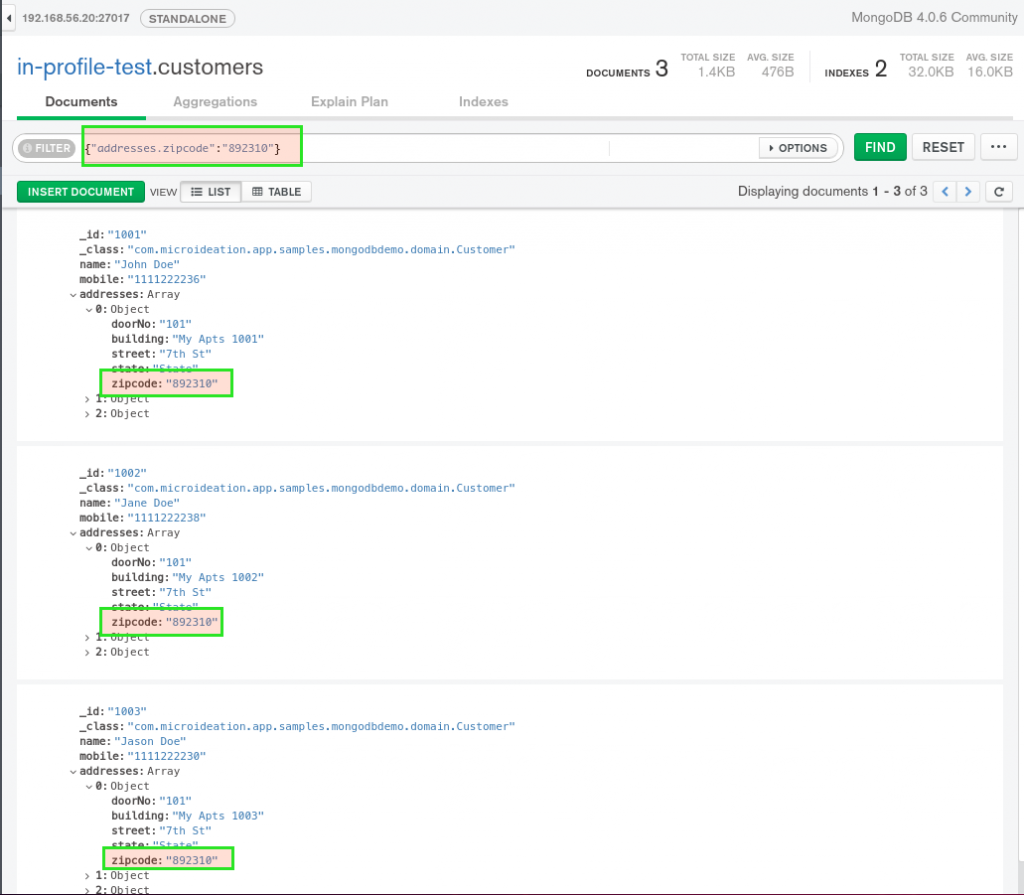
Note that the result will contain all documents that has got any of the embedded sub-document matching the field. Meaning, if there are 3 addresses are there and if any of the address has a matching field value, the document will returned.
Conclusion
We have seen the basic info on what MongoDB is and how we can execute some basic queries to get information from the Mongo database. We have also seen the setup of the Compass tool that we will be using extensively in the next part of the tutorial.
Part 2 Highlights
- Aggregation explanation
- Aggregation functions in Mongo
- Examples using Compass